In the next few blogs, I am going to be presenting examples of different chatbot methodologies in Python. In these examples we will be using a small list of keywords that would be in a typical chat for an auto dealership. In this blog, the chatbot being developed will be done using one of the most simplistic chatbot algorithms, yet you’ll see that even something as simple as this can be quite effective in communicating with a human.
In the first step, two empty dictionaries will be created, keywords and keywords_dict. With this methodology, it is necessary to put all of the keywords that would be part of a conversation into a grouping. The algorithm will then predict which category the user input falls into. As can be seen in the code below there are 5 groupings: greeting, sales, repair, oil, thanks. Note that ‘b’ is needed to denote a break.
keywords={}
keywords_dict={}
type(keywords)
keywords[‘greeting’]=[‘.*\\bhey\\b.*’,’.*\\bhi there\\b.*’,’.*\\bhello\\b.*’, ‘.*\\baloha\\b.*’,’.*\\bhi\\b.*’,’.*\\bwhats up\\b.*’],
keywords[‘sales’]=[‘.*\\blease\\b.*’,’.*\\bbuy\\b.*’,’.*\\btrade in\\b.*’,’.*\\btest drive\\b.*’, ‘.*\\bpurchase\\b.*’,’.*\\blook at\\b.*’,’.*\\bcheck out\\b.*’]
keywords[‘repair’]=[‘.*\\bsound\\b.*’,’.*\\bbroken\\b.*’,’.*\\btires\\b.*’,’.*\\bcheck up\\b.*’, ‘.*\\btune up\\b.*’,’.*\\bmuffler\\b.*’,’.*\\bmirror\\b.*’,’.*\\bnoise\\b.*’,’.*\\bsqueaking\\b.*’, ‘.*\\bbrakes\\b.*’]
keywords[‘oil’]=[‘.*\\boil\\b.*’,’.*\\boil change\\b.*’]
keywords[‘thanks’]=[‘.*\\bthanks\\b.*’,’.*\\bthank you\\b.*’,’.*\\bthx\\b.*’,’.*\\bty\\b.*’]
Our list of keywords above will be inserted into the empty keywords_dict dictionary. The ‘|’ is an OR statement for the dictionary.
for intent, keys in keywords.items():
keywords_dict[intent]=re.compile(‘|’.join(keys))
print (keywords_dict)
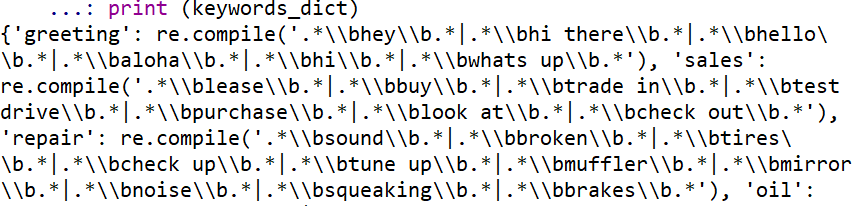
A dictionary of responses to the questions are created.
responses={
‘greeting’:”\n Hi there! How can I be of assistance?”,
‘sales’:”\n Let’s connect you to one of our sales representatives.”,
‘repair’:”\n Let’s schedule an appointment with our technicians.”,
‘oil’:”\n We have a deal on oil changes right now.”,
‘thanks’:”\n You’re welcome. Have a great day!”,
‘error’:”\n Sorry, I dont quite understand. Could you rephrase that?”}
The algorithm will now be created with a loop statement. The while (True) syntax will keep this running in the console until the user types ‘quit’ denoted by if user_input== ‘quit’. If it finds a keyword, then it will retrieve the corresponding intent from the dictionary. If there is an error, it will default to our error message “Sorry, I dont quite understand. Could you rephrase that?”. Finally print(responses[key]) will output the chatbot response.
print (“\n Welcome to our dealership! How may I help you? \n”)while (True):
user_input= input().lower()
f user_input== ‘quit’:
break
user_intent= None
for intent,pattern in keywords_dict.items():
if re.search(pattern, user_input):
user_intent= intent
key= ‘error’
if user_intent in responses:
key= user_intent
print(responses[key])
Now let’s take a look at the chatbot in action!
We just created a chatbot with a small amount of code and a handful of keywords and yet it is pretty useful at least in this situation. In the next blog post we’ll take a look at another methodology and see how it stacks up.